Essentials of Python for Data Science: A Starter Guide
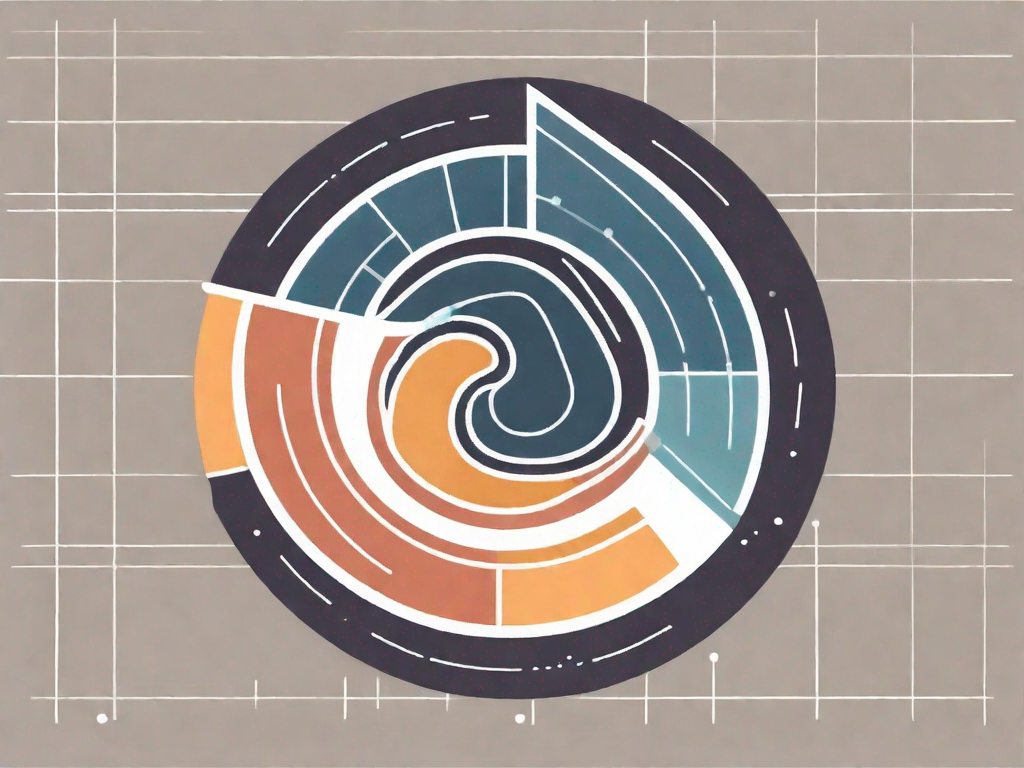
Python has become one of the most popular programming languages for data science, thanks to its simplicity, flexibility, and powerful libraries. Whether you are a beginner or an experienced programmer looking to dive into the world of data science, this starter guide will provide you with the essentials of Python and how it can be used for data science applications.
Understanding the Basics of Python
Introduction to Python Programming
Python is a versatile and beginner-friendly programming language that emphasizes readability and simplicity. It was first released in 1991 and has since gained a strong following among developers and data scientists alike. Python's syntax is designed to be intuitive and easy to understand, making it an ideal language for beginners to learn.
Python is an interpreted language, meaning that you can write and execute your code directly without the need for compiling. This makes it a highly interactive language, perfect for prototyping and exploring data. Additionally, Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming, providing programmers with a wide range of options when developing their applications.
When it comes to learning Python, it's important to understand the basics of the language. Python uses indentation to define blocks of code, which makes it easy to read and follow the flow of the program. It also has a dynamic type system, which means you don't need to declare the type of a variable before using it. Python also has a garbage collector that automatically manages memory, making it easier for developers to focus on writing code rather than worrying about memory management.
Key Features of Python
Python comes with a myriad of features that make it well-suited for data science applications. One of its key features is its extensive standard library, which provides a rich set of modules and functions for various purposes, including file input and output, networking, and data manipulation. In addition to the standard library, Python boasts a vast ecosystem of third-party libraries and packages specifically built for data science, such as NumPy, Pandas, and SciPy.
Python's standard library includes modules for working with regular expressions, handling dates and times, and parsing XML and JSON data. It also provides modules for working with databases, such as SQLite and PostgreSQL, making it easy to integrate Python with existing data storage systems. The standard library also includes modules for web development, allowing developers to build web applications using Python.
Another notable feature of Python is its cross-platform compatibility. Python programs can run on different operating systems, including Windows, macOS, and Linux, without requiring any modifications. This makes it convenient for data scientists who work on multiple platforms or collaborate with colleagues using different operating systems.
Python's cross-platform compatibility is further enhanced by its ability to interface with other programming languages. Python can be used as a scripting language to automate tasks in other applications, such as Microsoft Excel or Adobe Photoshop. It can also be used to extend the functionality of existing applications by writing Python modules in C or C++. This flexibility allows developers to leverage Python's strengths while still taking advantage of the capabilities of other programming languages.
Python for Data Science: Why It's a Perfect Match
Python's Versatility in Handling Data
One of the main reasons why Python is widely adopted in the field of data science is its ability to handle various types of data. Python provides built-in data structures such as lists, tuples, and dictionaries, which allow for efficient storage and manipulation of data. Additionally, Python's dynamic typing system enables developers to work with heterogeneous data, making it easier to represent and process complex datasets.
Python also offers powerful libraries for data cleaning, transformation, and analysis. Libraries such as Pandas provide easy-to-use data structures and data manipulation functions, allowing data scientists to efficiently explore and preprocess their datasets. Python's flexibility and expressiveness make it suitable for a wide range of data science tasks, from basic statistical analysis to machine learning and deep learning.
Python's Powerful Libraries for Data Science
Python's popularity in data science is largely driven by its extensive collection of libraries and packages tailored for data analysis and machine learning. NumPy, short for Numerical Python, provides efficient multi-dimensional arrays and mathematical functions that are essential for scientific computing. NumPy's array operations and broadcasting capabilities make it a powerful tool for performing complex computations on large datasets.
Pandas, on the other hand, offers high-level data structures and functions for data manipulation and analysis. Its DataFrame object, inspired by the data frame concept in R, allows for intuitive handling of structured data. Pandas supports various operations such as filtering, grouping, and merging, making it a go-to library for data cleaning and preparation.
Another noteworthy library is SciPy, which provides a wide range of scientific and numerical algorithms. From optimization and signal processing to statistical modeling and image processing, SciPy covers a broad spectrum of scientific computing tasks. When combined with other libraries such as Matplotlib for data visualization and Seaborn for statistical graphics, Python becomes a formidable toolset for data scientists.
Getting Started with Python for Data Science
Setting Up Your Python Environment
Before you embark on your data science journey with Python, it's crucial to set up your development environment. There are several options available, depending on your preferences and requirements. One of the most popular choices is Anaconda, a comprehensive Python distribution that comes bundled with a wide range of data science libraries, including NumPy, Pandas, and SciPy.
Once Anaconda is installed, you can create a virtual environment specific to your data science project. Virtual environments allow you to isolate your project's dependencies from the system Python installation, making it easier to manage and replicate your development environment. You can create a virtual environment using the command-line interface or the Anaconda Navigator graphical interface, whichever you find more convenient.
Basic Python Syntax for Data Science
Now that you have your Python environment set up, it's time to familiarize yourself with the basics of Python programming. Python syntax is known for its clarity and readability, which makes it an excellent language for beginners to learn. Here are a few essential concepts to get you started:
- Variables and Data Types: In Python, you can assign values to variables and perform operations on them. Python supports various data types, including integers, floats, strings, lists, tuples, and dictionaries.
- Control Flow: Python offers constructs such as if-else statements, loops, and function definitions to control the flow of execution in your programs.
- Functions and Modules: Python encourages a modular approach to programming. You can define your own functions to encapsulate reusable pieces of code, and import external modules to extend the functionality of your programs.
- Error Handling: Python provides mechanisms for handling exceptions and errors, allowing your programs to gracefully recover from unexpected situations.
Understanding these fundamental concepts will lay the foundation for your journey into data science with Python. With practice and exposure to real-world data science problems, you will gradually become proficient in Python programming and be able to tackle more advanced topics.
Diving Deeper into Python for Data Science
Advanced Python Concepts for Data Manipulation
As you gain more experience with Python for data science, you will likely encounter more advanced concepts and techniques for data manipulation. These include advanced NumPy operations such as broadcasting and vectorization, which can greatly improve the efficiency of your computations. You will also delve deeper into Pandas, exploring its advanced features for indexing, reshaping, and merging data.
In addition to NumPy and Pandas, you will explore other Python libraries and techniques for data analysis and visualization. This may include using interactive data visualization libraries like Plotly and Bokeh, as well as employing advanced statistical modeling techniques with libraries such as statsmodels and scikit-learn.
Data Visualization with Python
Data visualization is a critical aspect of data science, as it helps communicate insights and patterns in the data effectively. Python provides several libraries for creating visually appealing and informative visualizations. Matplotlib is a versatile library that offers a wide range of plots, including line plots, scatter plots, bar plots, and histograms. With Matplotlib, you can customize almost every aspect of your plots to suit your needs.
For more advanced and interactive visualizations, you can explore libraries like Seaborn, which is built on top of Matplotlib and offers high-level functions for creating attractive statistical graphics. Seaborn simplifies the process of creating complex visualizations by providing sensible defaults and easy-to-use APIs.
Python Libraries for Data Science
Introduction to NumPy and Pandas
NumPy and Pandas are two fundamental libraries in the Python ecosystem for data science. NumPy provides powerful tools for numerical computing, including multi-dimensional arrays, linear algebra operations, and random number generation. Its array operations and mathematical functions form the building blocks for many data science tasks.
Pandas, as mentioned earlier, offers high-level data structures and functions for data manipulation and analysis. Its DataFrame object is a two-dimensional table-like structure that is highly efficient and expressive. With Pandas, you can perform a wide range of data operations, such as filtering, aggregating, merging, and reshaping, all with a few lines of code.
Data Analysis with SciPy and Seaborn
In addition to NumPy and Pandas, SciPy and Seaborn are libraries that further enhance the capabilities of Python for data analysis and visualization. SciPy provides a rich set of functions and algorithms for scientific computing, covering areas such as optimization, interpolation, signal processing, and statistics. These tools are invaluable for tackling real-world data problems and conducting rigorous data analysis.
Seaborn, on the other hand, focuses on data visualization. It builds on top of Matplotlib and provides a high-level interface for creating aesthetically pleasing visualizations. Seaborn's extensive library of statistical graphics makes it easy to create informative plots, such as scatter plots, box plots, and heatmaps. These visualizations enable data scientists to explore patterns and relationships in their data, leading to valuable insights.
Conclusion
In summary, Python is a versatile and powerful programming language that is well-suited for data science applications. Its simplicity, flexibility, and extensive library ecosystem make it an excellent choice for beginners and experienced programmers alike. By mastering the essentials of Python and familiarizing yourself with the various libraries available, you can embark on an exciting journey into the world of data science and explore the vast possibilities that Python offers.